Create a safe and user-friendly login system.It is a crucial step in web development
Building a secure login system is one of the most important steps in building any web application. Whether you're creating a simple blog, an e-commerce platform, or a social network, you want to make sure that the user's credentials are protected and the authentication process is airtight. This is the least you can do for someone who's trusted their information to you. But how exactly do you build a secure login system in C#? Is C# capable of such a feat? Let’s walk through it together!
Why Secure Login Systems Matter
Imagine visiting a website, entering your password, and finding out later that someone else logged in to your account because the site didn’t take security seriously. Scary, right? It’s like locking the door to your house but using a plastic key.
What We’ll Cover:
- Setting up the Environment: Tools and frameworks you’ll need.
- Building the Login Page: Code walkthrough for a simple login form.
- Handling User Authentication: Validating credentials and creating user sessions.
- Security Best Practices: Protecting user data, handling passwords, and more.
Setting Up Your Environment
Before we write any code, you’ll need a few things in place:
- Visual Studio: The IDE where you’ll write your C# code.
- ASP.NET Core: The framework we’ll use to build the web application.
- SQL Server or SQLite: To store user information like usernames and passwords.
Building the Login Page
To begin, you’ll need a basic login form where users can enter their credentials. You have to build it yourself, but don't worry, I've got your back! Here’s a simple HTML form for your login page:
This form sends the username and password via a POST request to the /Account/Login
action in your controller.
Controller Action for Login
Next, we must write the code to handle the form submission by adding a Login method to our AccountController
. Here’s a simple example:
[HttpPost]
public IActionResult Login(string username, string password)
{
if (AuthenticateUser(username, password))
{
HttpContext.Session.SetString("Username", username);
return RedirectToAction("Index", "Home");
}
ViewBag.Error = "Invalid login credentials";
return View();
}
This example is just for demonstration. You should never hardcode usernames and passwords. It is like chiseling it into stone, making it harder if you later want to modify anything. Let’s look at how to handle authentication properly.
Handling User Authentication
To properly handle authentication, you’ll need to validate user credentials against a database and ensure that passwords are stored securely. One of the worst things you can do is store passwords in plain text. That accounts for nearly half the code vulnerabilities I've encountered throughout my professional life, so that is a very bad idea that you should avoid at all costs. Instead, use a secure hashing algorithm like BCrypt.
public string HashPassword(string password)
{
return BCrypt.Net.BCrypt.HashPassword(password);
}
public bool VerifyPassword(string password, string hashedPassword)
{
return BCrypt.Net.BCrypt.Verify(password, hashedPassword);
}
When the user tries to log in, you compare the entered password with the hashed version from your database. This authenticates the password in a safe and secure way.
Security Best Practices
Now that we’ve covered the basics, let’s talk about a few security best practices you should follow:
- Use HTTPS: Ensure your website uses HTTPS to encrypt communication between the server and the user’s browser.
- Rate Limit Login Attempts: Prevent brute-force attacks by limiting login attempts or using CAPTCHA after several failed attempts.
- Use Strong Password Policies: Enforce strong password requirements (e.g., minimum length, special characters).
- Session Timeout: Set session timeouts to automatically log users out after a period of inactivity.
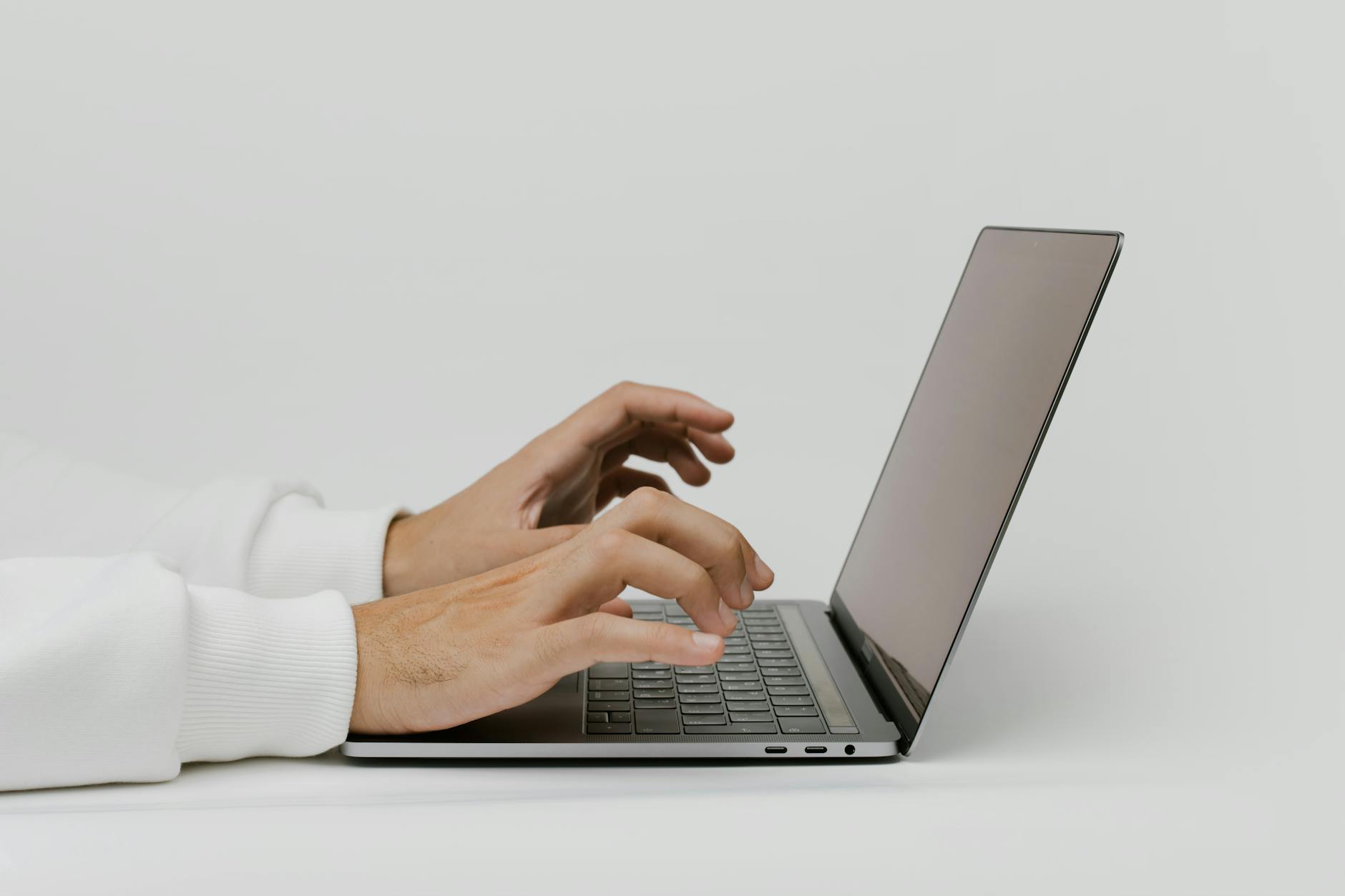
Building a secure login system is a crucial part of any web application. By following the steps in this guide, you’ll not only create a functional login page but also ensure that it’s secure. Remember to hash passwords, validate input, and manage sessions correctly, and you'll have a modern, safe and pleasant site.
If you're building your own login system, I encourage you to implement the security practices discussed here, to avoid any unwanted breach of cyber-security. Have questions or need more clarification? Drop a comment below—I’d love to help!