When you're making a game, one of the first things you'll need to figure out is how to handle player input. This means knowing how to capture and respond to what the player is doing—whether that's pressing a key on the keyboard, clicking the mouse, or using a game controller. In this guide, I’ll show you how to handle input in C# and how you can use it in your games, whether you’re building from scratch or using Unity.
By the end, you’ll understand how to capture input from different devices, process that input, and apply it to control things like player movement. I’ll also give you best practices and some common mistakes to avoid. Let’s get started.
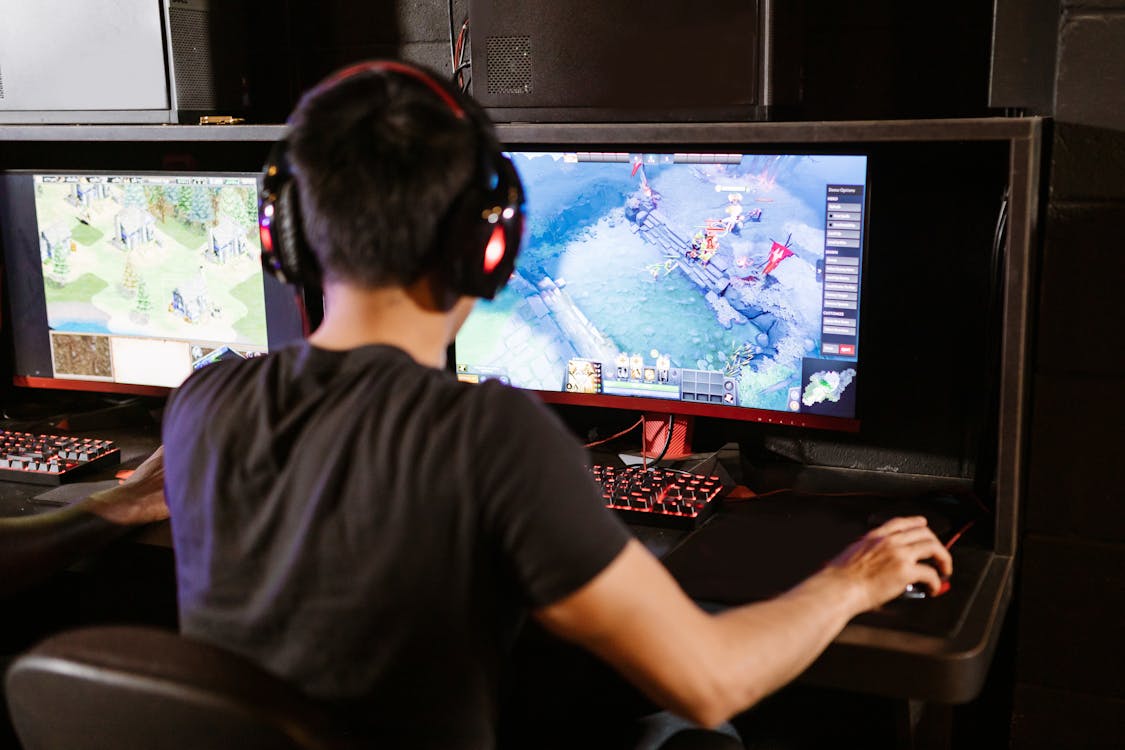
1. Basics of Input Handling in C#
Input handling in C# starts by listening for what the player does—whether they press a key, click the mouse, or use a gamepad. In Unity, we use the Input class to capture player actions. Let me show you the simplest way to detect when a player presses a key.
Detecting Key Presses
void Update()
{
if (Input.GetKeyDown(KeyCode.Space))
{
Debug.Log("Space key was pressed");
}
}
In this code, the Update() function checks every frame if the player presses the spacebar. When it’s pressed, a message appears in the console.
Why Use Update()?
In Unity, Update() runs once per frame, which means it’s perfect for detecting input because the player could press a key at any time, and you need to check for it continuously.
Polling vs. Events
Polling is what we’re doing here—checking every frame whether something happened. In some other game engines or setups, you might use events, where you tell the game to “listen” for a specific input and only react when that input happens. Unity mainly uses polling for input handling, so we’ll stick with that.
2. Handling Keyboard Input
Most games use the keyboard for movement or actions, so let’s look at how to handle basic keyboard input in C#. I’ll show you how to move a character using the arrow keys.
Moving a Character with Arrow Keys
public float moveSpeed = 5f;
void Update()
{
float moveX = Input.GetAxis("Horizontal") * moveSpeed * Time.deltaTime;
float moveY = Input.GetAxis("Vertical") * moveSpeed * Time.deltaTime;
transform.Translate(new Vector3(moveX, moveY, 0));
}
Here, we use Unity’s Input.GetAxis() to get the input from the arrow keys or WASD keys (these are mapped by default in Unity). Horizontal returns a value between -1 and 1, where -1 is the left arrow (or "A") and 1 is the right arrow (or "D"). Similarly, Vertical returns -1 for down or "S" and 1 for up or "W".
We then multiply these values by moveSpeed (which controls how fast the player moves) and Time.deltaTime (which makes sure the movement speed is consistent, no matter the frame rate). Finally, we use transform.Translate() to move the character in the X and Y directions.
Customizing Input Mappings
You might want to let the player customize their controls. In Unity, you can do this through the Input Manager (Edit > Project Settings > Input Manager). You can create new axes and name them however you like (e.g., “Jump” or “Sprint”). Then, you can refer to them in your code like this:
if (Input.GetButtonDown("Jump"))
{
// Perform jump action
}
This allows for more flexible control schemes without hardcoding specific keys.
3. Handling Mouse Input
Mouse input is commonly used for aiming, shooting, or controlling the camera. Let’s go over how to detect mouse clicks and movements.
Detecting Mouse Clicks
void Update()
{
if (Input.GetMouseButtonDown(0)) // Left-click
{
Debug.Log("Left mouse button clicked");
}
}
This simple code detects when the player presses the left mouse button (button 0). You can replace 0 with 1 for the right mouse button or 2 for the middle button.
Tracking Mouse Movement
void Update()
{
float mouseX = Input.GetAxis("Mouse X");
float mouseY = Input.GetAxis("Mouse Y");
transform.Rotate(Vector3.up, mouseX);
transform.Rotate(Vector3.left, mouseY);
}
Here, Input.GetAxis("Mouse X") and Input.GetAxis("Mouse Y") give us the movement of the mouse. This is commonly used in first-person games to control the camera’s direction.

4. Handling Gamepad/Controller Input
Many players use gamepads or controllers, especially on consoles. Unity makes it pretty easy to handle gamepad input using the same Input class.
Moving with a Gamepad
void Update()
{
float moveX = Input.GetAxis("Horizontal") * moveSpeed * Time.deltaTime;
float moveY = Input.GetAxis("Vertical") * moveSpeed * Time.deltaTime;
transform.Translate(new Vector3(moveX, moveY, 0));
}
It’s the same code as for keyboard input! Unity automatically maps the gamepad’s left stick to the same "Horizontal" and "Vertical" axes. That means you don’t need separate code for keyboard and gamepad input—Unity handles it all in the background.
Gamepad Buttons
if (Input.GetButtonDown("Fire1"))
{
// Perform action like shooting
}
By default, "Fire1" is mapped to the gamepad’s A button (or X button on a PlayStation controller).
5. Advanced Input Handling Techniques
Once you’ve mastered the basics, you might want to add some advanced features to your input handling.
Input Smoothing
To make movement feel smoother, especially with gamepads, you can apply input smoothing. Instead of reacting immediately to input, you can gradually move the player toward the desired direction:
Vector3 velocity = Vector3.zero;
void Update()
{
float moveX = Input.GetAxis("Horizontal");
float moveY = Input.GetAxis("Vertical");
Vector3 targetPosition = new Vector3(moveX, moveY, 0);
transform.position = Vector3.SmoothDamp(transform.position, targetPosition, ref velocity, 0.3f);
}
SmoothDamp makes sure the movement accelerates and decelerates smoothly, making the player’s movement feel more natural.
Input Buffering
Sometimes players press a button just before the game is ready to respond (like right before a jump). Input buffering lets you "remember" these inputs for a short time, so the game still responds even if the timing is slightly off.
float lastJumpTime = -1f;
float bufferTime = 0.2f;
void Update()
{
if (Input.GetButtonDown("Jump"))
{
lastJumpTime = Time.time;
}
if (Time.time - lastJumpTime <= bufferTime)
{
// Perform jump action
lastJumpTime = -1f;
}
}
This makes sure that even if the player presses the jump button slightly before the game is ready, the jump will still happen within the buffer window.
6. Common Mistakes and Best Practices
When handling input, it's easy to run into a few common mistakes. Let me help you avoid them:
Hardcoding Inputs
It’s tempting to hardcode specific keys directly into your game logic (like always checking for the spacebar to jump). This works for small projects, but it’s not scalable. Instead, use Unity’s Input Manager to set up customizable controls.
Input Handling and Game Logic
Keep your input handling code separate from your gameplay logic. This makes your code more modular and easier to maintain. You can achieve this by having a dedicated input handler that passes commands to your gameplay code.
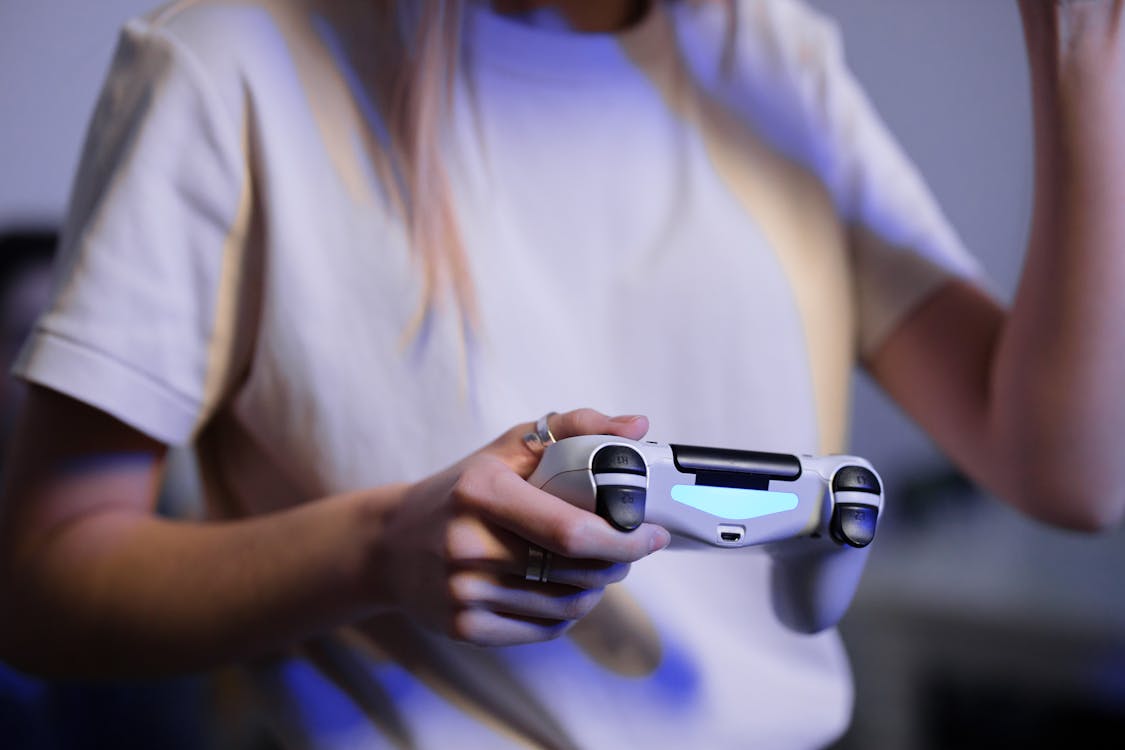
Handling input in C# is one of the core skills you’ll need to master in game development. Whether you’re working with keyboards, mice, or gamepads, it’s all about capturing the player’s actions and translating them into gameplay.
Start with the basics—like detecting key presses or mouse clicks—then gradually build up to more advanced techniques like input smoothing and buffering. Remember, clean and scalable input code will make your game easier to maintain and adapt as your project grows.
Now it’s your turn! Go ahead and try out these techniques in your own projects. You’ll be amazed at how responsive and polished your game will feel when you handle input correctly.